Table of Contents
Coding problems can be daunting and frustrating for programmers of all levels. However, with the right strategies and approach, you can effectively solve coding problems and improve your problem-solving skills. In this article, we will provide you with some practical tips and techniques to help you tackle coding problems with confidence.
To begin with, understanding the problem is crucial. Before you start writing any code, read the problem statement carefully and identify the input, output, and any constraints or edge cases. Once you have a clear understanding of the problem, you can develop a strategy to solve it. This involves breaking down the problem into smaller steps and thinking about potential algorithms or data structures that could be used to solve it. Writing pseudocode or working through examples can also be helpful in solidifying your understanding of the problem and the solution approach.
Implementing the solution is the next step, where you write the code in your preferred programming language, following best practices and considering edge cases. Testing and debugging your solution is also crucial to ensure it works as expected. Once you have a working solution, it’s important to analyze its time and space complexity and explore ways to optimize it if necessary. Consistent practice is key to improving problem-solving skills, so regularly attempting problems on coding platforms and learning from others’ solutions can be very helpful.
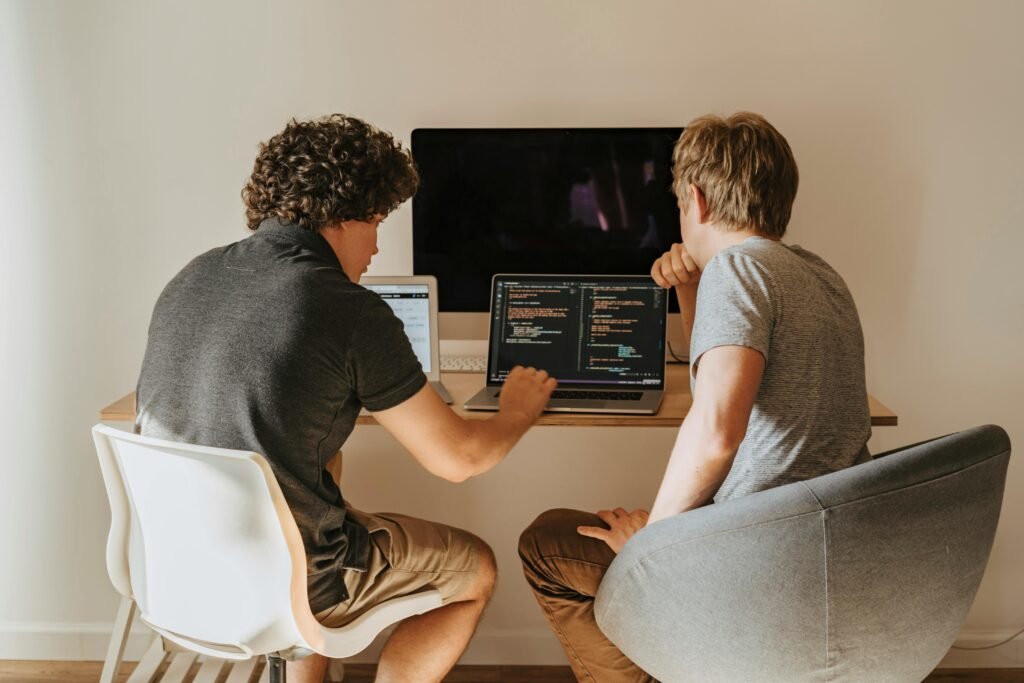
Key Takeaways
- Understanding the problem and developing a strategy are crucial for solving coding problems effectively.
- Writing pseudocode and working through examples can help solidify your understanding of the problem and the solution approach.
- Consistent practice, testing and debugging, and engaging with the coding community can help improve your problem-solving skills.
Understanding the Problem
When faced with a coding problem, the first step is to carefully read and understand the problem statement. This involves analyzing the problem statement, identifying inputs and outputs, and considering constraints and edge cases.
Analyzing the Problem Statement
To effectively understand the problem, you should read the problem statement carefully and break it down into smaller parts. This can be done by identifying the key requirements of the problem, such as the expected inputs and outputs, and any constraints or edge cases that may need to be considered.
Identifying Inputs and Outputs
Once you have analyzed the problem statement, the next step is to identify the inputs and expected outputs of the problem. This involves understanding the data types and formats of the inputs and outputs, and any specific requirements or restrictions on their values.
Considering Constraints and Edge Cases
In addition to understanding the inputs and outputs, it is important to consider any constraints or edge cases that may impact the solution approach. This could include limitations on the size or range of the inputs, as well as any unusual or unexpected scenarios that may need to be accounted for.
By taking the time to thoroughly understand the problem statement, identify the inputs and outputs, and consider any constraints or edge cases, you will be better equipped to develop an effective strategy for solving the coding problem.
Strategizing the Solution
When faced with a coding problem, it’s important to develop a strategy before diving into writing code. Here are some key steps to consider when strategizing your solution.
Breaking Down into Subproblems
One effective strategy is to break down the problem into smaller subproblems. This can make the problem more manageable and easier to solve. Start by identifying the key inputs and outputs of the problem, as well as any edge cases or constraints. Then, think about how you can break the problem down into smaller subproblems that can be solved individually.
Selecting Appropriate Algorithms
Once you have broken down the problem into subproblems, it’s important to think about potential algorithms that could be used to solve each subproblem. Consider the time and space complexity of each algorithm, as well as any trade-offs between them. Choose the algorithm that is most appropriate for the problem at hand.

Choosing Efficient Data Structures
In addition to selecting appropriate algorithms, it’s also important to choose efficient data structures. Consider the size of the input data and the operations that will be performed on it. Choose a data structure that can handle the input size and optimize for the operations that will be performed most frequently.
When solving coding problems effectively, breaking them into subproblems, choosing appropriate algorithms, and using efficient data structures are essential steps. Before writing actual code, remember to create pseudocode or work through examples, and thoroughly test and debug your solution. Consistent practice and a willingness to learn from others can help you improve your problem-solving skills and become a more effective coder.
Pseudocode and Examples
Writing Pseudocode
Before diving into writing the actual code, it can be helpful to write pseudocode to map out your solution approach. Pseudocode is a high-level description of the steps involved in solving a problem, written in a language-agnostic way. It can help you clarify your thinking and identify any potential issues with your approach before you start writing code.
When writing pseudocode, focus on breaking down the problem into smaller subproblems. Identify the inputs, outputs, and any constraints or edge cases. Then, think about potential algorithms or data structures that could be used to solve the problem. Write out the steps involved in your solution approach, using clear and concise language.
Here’s an example of pseudocode for solving the classic FizzBuzz problem:
for i in range(1, n+1):
if i % 3 == 0 and i % 5 == 0:
print("FizzBuzz")
elif i % 3 == 0:
print("Fizz")
elif i % 5 == 0:
print("Buzz")
else:
print(i)
Working Through Examples
In addition to writing pseudocode, working through examples can be a helpful way to solidify your understanding of the problem and the solution approach. Start by selecting a few input cases, including edge cases, and manually work through the steps involved in your solution approach.
For example, let’s say you’re working on a problem that involves sorting an array of integers in ascending order. Here’s an example of working through an input case:
1. Identification of inputs and outputs: Input array - [2, 1, 4, 1, 5, 10, 2, 6, 5, 8]; Output array - [1, 1, 2, 2, 4, 5, 5, 6, 8, 10].
2. Strategy development: Consider using a sorting algorithm like quicksort or mergesort.
3. Writing pseudocode: Write out the steps involved in the selected algorithm using pseudocode.
4. Example: Manually sort the input array using the pseudocode to ensure the algorithm is correct.
5. Implementation of the solution: Write the code in your preferred programming language, following best practices and considering edge cases.
6. Testing and debugging: Test the solution with various input cases, including edge cases, and debug any issues that arise.
7. Optimization: Analyze the time and space complexity of your solution and explore ways to optimize it if necessary.
These examples can help you gain a deeper understanding of the problem and the solution approach, and identify any potential issues with your strategy before you start writing code.
Implementation of Code
Once you have developed a strategy for solving a coding problem, the next step is to implement your solution. This involves writing code in your preferred programming language, following best practices, and considering edge cases.
Writing the Initial Code
When writing your initial code, it is important to follow the strategy you developed and consider the input, output, and any constraints or edge cases. Start by writing the basic structure of the code, such as defining variables or functions, and then gradually add more complexity to the code.
Applying Coding Standards
To ensure that your code is readable and maintainable, it is important to follow coding standards. This includes using consistent naming conventions, formatting the code properly, and avoiding redundant or unnecessary code. By following coding standards, your code will be easier to understand and modify in the future.
Incorporating Comments for Clarity
Adding comments to your code is essential for clarity and understanding. Comments provide context and explanations for the code, making it easier for others to understand and modify. When adding comments, be sure to explain the purpose of the code, any assumptions or limitations, and any potential issues or edge cases.
Remember to test and debug your code, optimize it if necessary, and continue practicing to improve your problem-solving skills.
Testing and Debugging
When you have implemented your solution, it is important to test it thoroughly to ensure that it works as expected. Testing involves running your code with various input cases, including edge cases, and verifying that it produces the correct output.
Developing Test Cases
To develop effective test cases, you should consider the input and output requirements of the problem, as well as any constraints or edge cases. You can use a table to organize your test cases, with columns for the input, expected output, and actual output. This can help you keep track of which test cases have passed and which have failed.
It is also important to consider the time and space complexity of your solution when developing test cases. For example, you might want to test your solution with large input sizes to ensure that it can handle the maximum input size specified in the problem statement.
Identifying and Fixing Errors
When testing your code, you may encounter errors or bugs that prevent it from producing the correct output. This is where debugging comes in.
Common mistakes when debugging include not reading error messages carefully, assuming that your code is correct without verifying it, and not considering all possible edge cases. To avoid these mistakes, take the time to carefully read error messages and use print statements or a debugger to step through your code and verify that it is working as expected.
Once you have identified an error, you can use various techniques to fix it. These include checking for syntax errors, logic errors, and off-by-one errors, as well as verifying that your code is correctly handling edge cases.
Ensure that your code is correct and efficient, and that it meets the requirements of the problem statement.
Optimization and Refinement
Analyzing Complexity
Once you have a working solution to a coding problem, it’s important to analyze its time and space complexity. This will help you identify any inefficiencies in your code and explore ways to optimize it. Time complexity refers to the amount of time it takes for your algorithm to run as the input size grows. Space complexity refers to the amount of memory your algorithm uses as the input size grows.
To analyze time complexity, you can use Big O notation, which expresses the upper bound of the worst-case scenario for your algorithm’s runtime. For example, an algorithm with a time complexity of O(n) means that its runtime grows linearly with the input size. An algorithm with a time complexity of O(n^2) means that its runtime grows quadratically with the input size.
To analyze space complexity, you can use the same notation to express the upper bound of the worst-case scenario for your algorithm’s memory usage. For example, an algorithm with a space complexity of O(n) means that its memory usage grows linearly with the input size. An algorithm with a space complexity of O(n^2) means that its memory usage grows quadratically with the input size.
Applying Optimization Techniques
Once you have analyzed your algorithm’s complexity, you can explore ways to optimize it. There are many techniques you can use to optimize your code, including:
- Memoization: Memoization is a technique that involves storing the results of expensive function calls and returning the cached result when the same inputs occur again. This can greatly improve the runtime of recursive algorithms.
- Dynamic programming: Dynamic programming is a technique that involves breaking down a problem into smaller subproblems and solving each subproblem only once. This can greatly improve the runtime of algorithms that involve repeated calculations.
- Greedy algorithms: Greedy algorithms are algorithms that make the locally optimal choice at each step in the hope of finding a global optimum. This can greatly improve the runtime of algorithms that involve optimization problems.
- Data structure optimization: Choosing the right data structure for your problem can greatly improve the runtime and space complexity of your algorithm. For example, using a hash table instead of an array can greatly improve lookup time.
When optimizing your code, it’s important to balance runtime and space complexity. Sometimes optimizing for one can come at the expense of the other. It’s also important to consider edge cases and test your optimized solution thoroughly to ensure it still works correctly.
By refining and optimizing your code, you can improve your problem-solving skills and become a more efficient and effective coder.
Continuous Practice and Growth
To become a proficient problem solver, it’s essential to engage in regular practice. Consistent practice allows you to build your problem-solving skills and develop your ability to think critically. By practicing regularly, you can also stay up-to-date with the latest trends and techniques in the coding world.
Engaging in Regular Practice
One of the best ways to practice your coding skills is by using online coding platforms like LeetCode and HackerRank. These platforms offer a wide range of coding problems that you can solve to hone your skills. You can also participate in coding contests and challenges to test your skills against other coders.
Another way to practice is by working on personal coding projects. This allows you to work on problems that interest you and apply the skills you’ve learned to real-world scenarios. You can also collaborate with other coders on open-source projects to learn from their expertise and gain valuable feedback.
Learning from Peer Review
After solving a problem, it’s important to review your solution and learn from your mistakes. You can also learn from the solutions provided by other coders on the platform. This can help you gain new insights and techniques that you can apply to future problems.
Participating in discussions with other coders can also provide valuable feedback and insights. You can ask questions, share your solutions, and learn from the experiences of other coders. This can help you identify areas where you need to improve and gain new perspectives on problem-solving.
In conclusion, continuous practice and growth are essential to becoming a proficient problem solver. By engaging in regular practice, learning from peer review, and participating in discussions, you can build your problem-solving skills, stay up-to-date with the latest trends and techniques, and gain valuable feedback.
Community Engagement and Learning
Engaging with the coding community can be an excellent way to learn new concepts and techniques, build your coding skills, and gain confidence in your abilities. Here are some ways to participate in coding communities:
Participating in Coding Communities
Participating in coding communities can provide valuable insights and feedback, as well as opportunities to collaborate with other coders. You can join coding communities on platforms like LeetCode, HackerRank, or GitHub. Here are some ways to engage with these communities:
- Participate in discussions: Engage with other coders by asking questions, sharing your solutions, and providing feedback on others’ solutions.
- Attend meetups or hackathons: Attend local meetups or hackathons to meet other coders and work on coding challenges together.
- Contribute to open-source projects: Contribute to open-source projects on GitHub, which can help you build your coding skills and gain experience working on real-world projects.
Exploring New Concepts and Techniques
Exploring new concepts and techniques can help you improve your coding skills and prepare for coding interviews or technical interviews. Here are some ways to explore new concepts and techniques:
- Read coding blogs or books: Read coding blogs or books to learn about new concepts and techniques in coding.
- Take online courses: Take online courses on platforms like Coursera, edX, or Udemy to learn new coding concepts and techniques.
- Solve coding challenges: Solve coding challenges on platforms like LeetCode or HackerRank to practice applying new concepts and techniques.
By participating in coding communities and exploring new concepts and techniques, you can build your coding skills, prepare for coding interviews or technical interviews, and gain confidence in your abilities.
Frequently Asked Questions
What are the best practices for solving coding problems effectively?
To solve coding problems effectively, it is important to first understand the problem statement and identify the input, output, and constraints or edge cases. Then, break down the problem into smaller steps and think about potential algorithms or data structures that could be used to solve it. Before writing the actual code, it can be helpful to write pseudocode or work through examples to solidify your understanding of the problem and the solution approach. Once you have a working solution, test it with various input cases, including edge cases, and debug any issues that arise. Finally, analyze the time and space complexity of your solution and explore ways to optimize it if necessary.
Can you provide examples of problem-solving strategies used in programming?
Some common problem-solving strategies used in programming include brute force, greedy algorithms, dynamic programming, divide and conquer, and backtracking. Brute force involves trying every possible solution until a correct one is found. Greedy algorithms make the locally optimal choice at each step to find a globally optimal solution. Dynamic programming involves breaking down a problem into smaller subproblems and solving each subproblem only once. Divide and conquer involves breaking down a problem into smaller subproblems and solving each subproblem independently. Backtracking involves exploring all possible solutions and undoing choices that do not lead to a correct solution.
How can one improve their coding problem-solving skills through practice?
Consistent practice is key to improving coding problem-solving skills. Regularly attempt problems on coding platforms like LeetCode, HackerRank, or others to build your expertise. After solving a problem, review the solutions provided by others or the platform itself. This can help you learn new techniques and approaches. Engage with the coding community on these platforms by participating in discussions, asking questions, and sharing your solutions. This can provide valuable insights and feedback.
What are the steps involved in the problem-solving process specific to coding?
The problem-solving process specific to coding involves understanding the problem statement, developing a strategy, writing pseudocode or examples, implementing the solution, testing and debugging, optimizing the solution, and practicing regularly. It is important to consistently practice and engage with the coding community to improve problem-solving skills.
How do online platforms for solving coding problems help in improving programming skills?
Online platforms for solving coding problems provide a variety of problems to solve, ranging from easy to difficult. These platforms also provide solutions to the problems, allowing users to learn new techniques and approaches. Additionally, these platforms often have a community of coders who engage in discussions, ask questions, and share solutions, providing valuable insights and feedback.
What are some common pitfalls to avoid when attempting to solve coding problems?
Some common pitfalls to avoid when attempting to solve coding problems include not understanding the problem statement fully, not considering edge cases or constraints, not breaking down the problem into smaller steps, not testing the solution with various input cases, and not analyzing the time and space complexity of the solution. It is important to take the time to fully understand the problem and consider all aspects before attempting to solve it.
Conclusion
In conclusion, becoming a great problem-solver in coding requires understanding problems, coming up with a plan, writing good code, testing and fixing any issues, and always learning and practicing more. Keep at it and you’ll become a pro!
More information
Related Content
Algorithms and Data Structures: The Foundations of Programming
Functional vs. Object-Oriented Programming: 5 Crucial and Exciting Differences
Unlock Your Potential: Continuous Learning for Busy Adults – Mastering New Skills After 40